what command is used to change a string into a number?
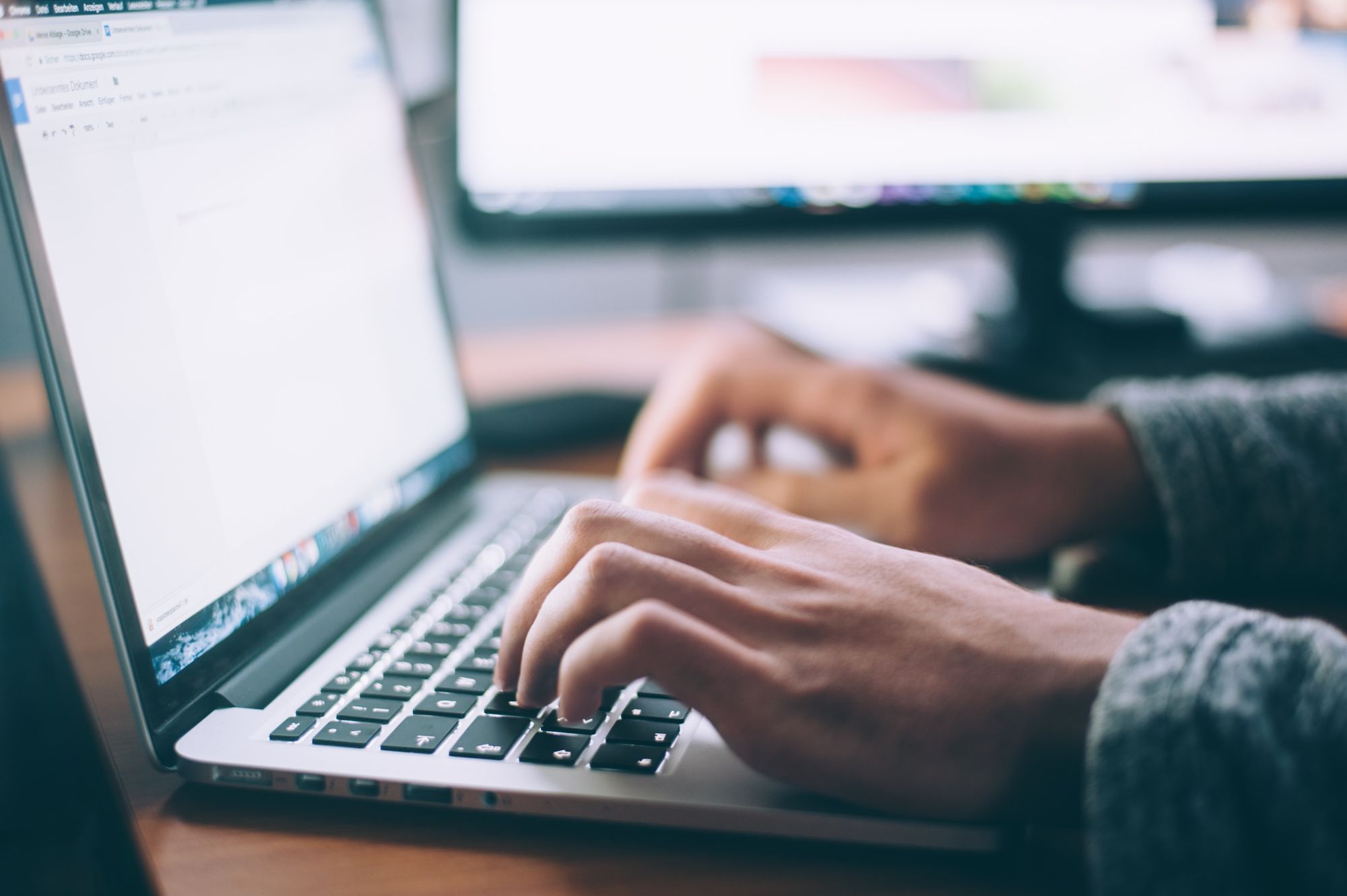
When y'all're programming, yous'll often need to switch betwixt data types.
The ability to convert one information type to another gives you lot great flexibility when working with data.
At that place are dissimilar built-in ways to catechumen, or bandage, types in the Python programming language.
In this article, you'll learn how to convert a string to an integer.
Let's become started!
Data types in Python
Python supports a variety of data types.
Data types are used for specifing, representing, and caregorizing the different kinds of data that exist and are used in figurer programs.
Also, different operations are available with different types of data – 1 operation available in one data blazon is often not bachelor in another.
One case of a data type is strings.
Strings are sequences of characters that are used for carrying textual information.
They are enclosed in single or double quotation marks, like so:
fave_phrase = "Hello globe!" #Hello world! is a string,enclosed in double quotation marks
Ints, or integers, are whole numbers.
They are used to represent numerical data, and y'all can do any mathematical operation (such as add-on, subtraction, multiplication, and division) when working with integers.
Integers are not enclosed in unmarried or double quotation marks.
fave_number = 7 #7 is an int #"7" would not be an int merely a string, despite it being a number. #This is because of the quotation marks surrounding it
Data type conversion
Sometimes when you're storing information, or when you receive input from a user in ane type, you'll demand to manipulate and perform different kinds of operations on that data.
Since each information type can exist manipulated in dissimilar ways, this often means that you'll need to convert it.
Converting 1 information type to another is also chosen type casting or type conversion. Many languages offer born cast operators to do simply that – to explicitely convert 1 type to another.
How to convert a cord to an integer in Python
To convert, or cast, a string to an integer in Python, you lot use the int()
built-in function.
The part takes in as a parameter the initial string you want to convert, and returns the integer equivalent of the value you passed.
The full general syntax looks something like this: int("str")
.
Allow's take the post-obit example, where there is the string version of a number:
#cord version of the number seven print("7") #check the data type with type() method print(type("7")) #output #vii #<class 'str'>
To convert the string version of the number to the integer equivalent, you utilise the int()
office, like so:
#convert string to int information type print(int("7")) #check the data type with type() method print(type(int("7"))) #output #7 #<class 'int'>
A practical instance of converting a cord to an int
Say you lot want to calculate the age of a user. You do this by receiving input from them. That input volition always be in string format.
So, fifty-fifty if they type in a number, that number will exist of <class 'str'>
.
If you desire to then perform mathematical operations on that input, such as subtract that input from another number, you will get an error because y'all can't carry out mathematical operations on strings.
Cheque out the instance beneath to meet this in action:
current_year = 2021 #ask user to input their twelvemonth of nativity user_birth_year_input = input("What yr were you built-in? ") #subtract the current year from the twelvemonth the user filled in user_age = current_year - user_birth_year_input print(user_age) #output #What twelvemonth were yous born? 1993 #Traceback (most contempo telephone call last): # File "demo.py", line 9, in <module> # user_age = current_year - user_birth_year_input #TypeError: unsupported operand type(s) for -: 'int' and 'str'
The error mentions that subtraction can't be performed between an int and a cord.
Yous can bank check the data type of the input by using the type()
method:
current_year = 2021 #enquire user to input their year of nascence user_birth_year_input = input("What year were y'all born? ") impress(type(user_birth_year_input)) #output #What yr were you born? 1993 #<grade 'str'>
The mode around this and to avoid errors is to convert the user input to an integer and store it inside a new variable:
current_year = 2021 #ask user to input their yr of nativity user_birth_year_input = input("What year were you lot born? ") #convert the raw user input to an int using the int() office and store in new variable user_birth_year = int(user_birth_year_input) #decrease the current twelvemonth from the converted user input user_age = current_year - user_birth_year print(user_age) #output #What year were you lot born? 1993 #28
Conclusion
And there you have it - you now know how to convert strings to integers in Python!
If yous desire to larn more about the Python programming linguistic communication, freeCodeCamp has a Python Certification to get y'all started.
You'll get-go with the fundamentals and progress to more advance topics like data structures and relational databases. In the cease, you'll build five projects to practice what you learned.
Thanks for reading and happy coding!
Acquire to code for free. freeCodeCamp'due south open source curriculum has helped more than 40,000 people become jobs as developers. Get started
Source: https://www.freecodecamp.org/news/python-convert-string-to-int-how-to-cast-a-string-in-python/